You don’t need anotherbilling systemto ship fasternew pricing modelsAI monetizationcredits & top-upsadd-ons & bundlesusage-based pricinglocalized pricing
There’s a better way.
The first scalable monetization platform,
for the modern billing stack. Eliminate risk. Retain focus. Power more pricing and packaging options with less code.
Trusted by


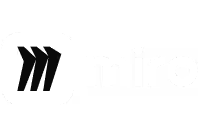
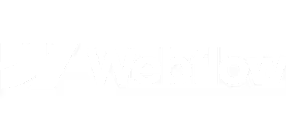

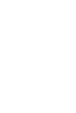
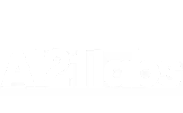
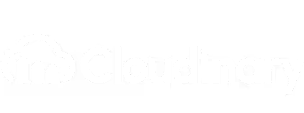
What is a monetization platform?
A monetization platform is a standalone middleware that sits between your application and your business applications, as part of the modern enterprise billing stack.
Stigg unifies all the APIs and abstractions billing and platform engineers had to build and maintain in-house otherwise. Acting as your centralized source of truth, with a highly scalable and flexible entitlements management, rolling out any pricing and packaging change is now a self-service, risk-free, exercise.
Modeling
Consolidate pricing and packaging models to create a single source of truth for product catalog definitions. Streamline workflows with flexible building blocks.
& Provisioning
Centralize entitlements and provisioning, offering flexible access management, streamlined operations, and customer experiences for growth at scale.
Metering
Meter usage in real-time, compute metrics, and ingest events at large scale. Enable usage-based pricing and consumption limits, while ensuring billing accuracy and cost efficiency.
Management
Robust subscription management capabilities to streamline your billing processes and enhance customer experiences for both self-service and direct channels.
Linage &
Migrations
Maintain consistency with plan versions, automate price migrations gracefully. Adapt swiftly to market changes with minimal disruption for customers.
UIs
Easily embed customizable widgets that enhance user interfaces and enrich user experience, ensuring a fluid and cohesive user journey. Â
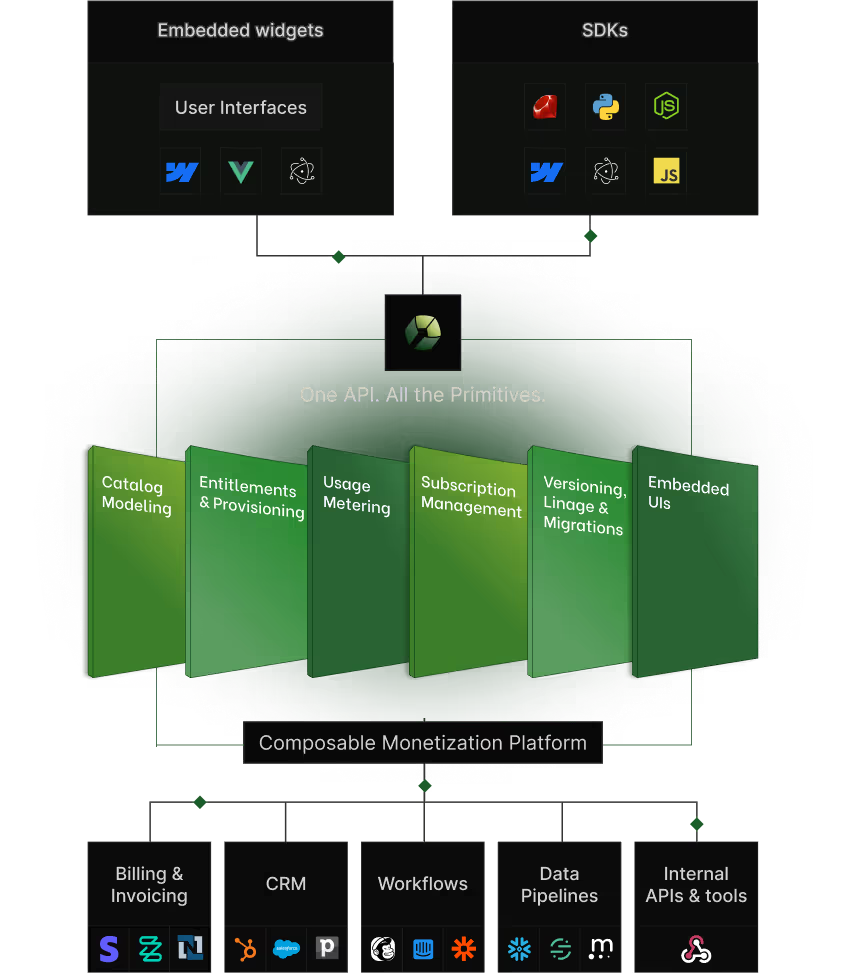
Why Stigg?
What are Entitlements?
Stigg’s Entitlements give engineers fine-grained control over what can be packaged and priced separately. You can set limits and govern your customers’ commercial permissions at the feature level, abstracting away complex billing concepts from your code.
‍
Entitlements are the modern way to software monetization and truly flexible hybrid pricing.
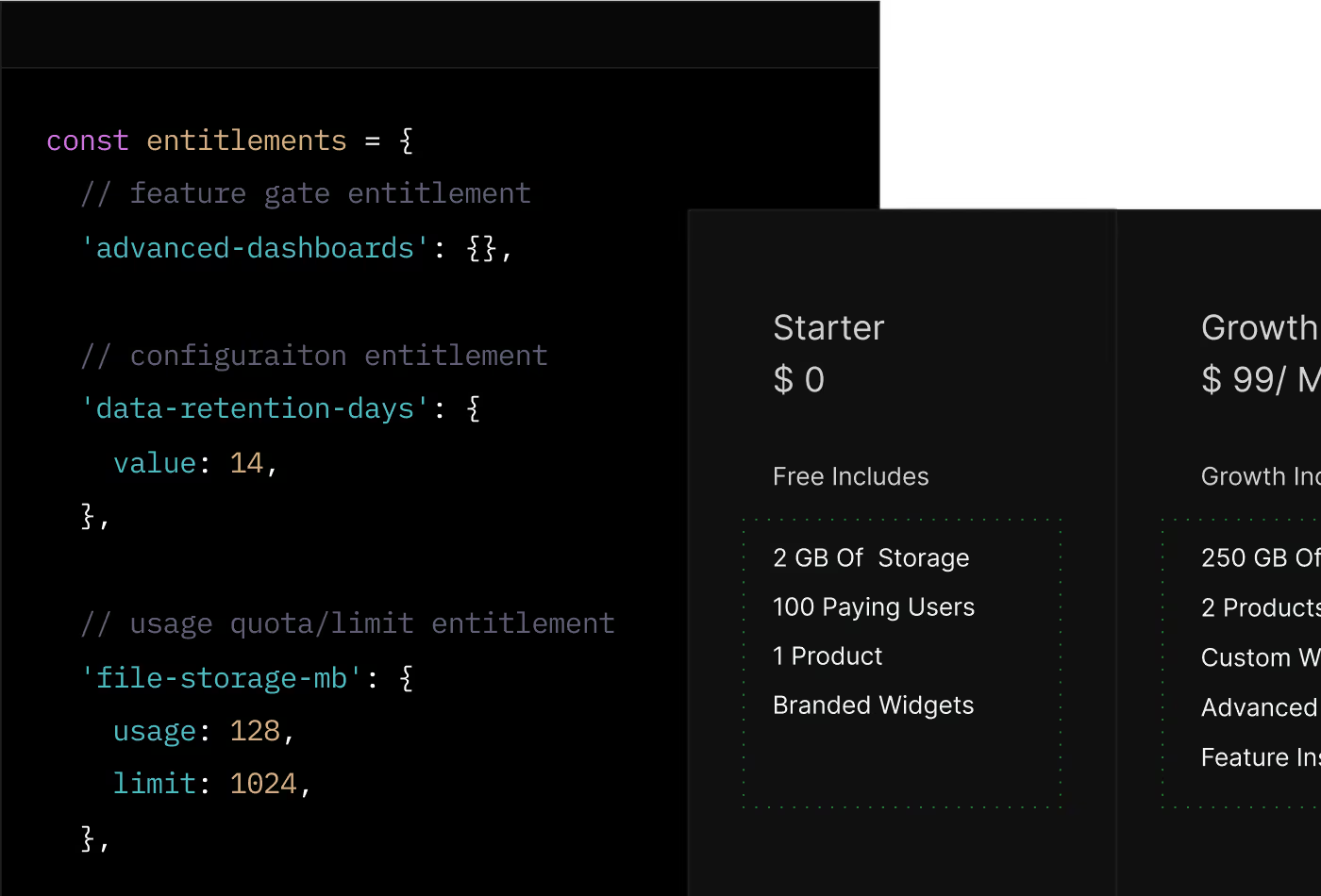
One API. All the Pricing and Packaging options.
Move from
“It will take 2 quarters”
to “We can support it today”.
Stigg replaces augments your existing billing stack
Get started with Stigg the way you want.
SDKs frameworks
Sidecar
Use your own DB
The most powerful entitlement infrastructure
 you will never build
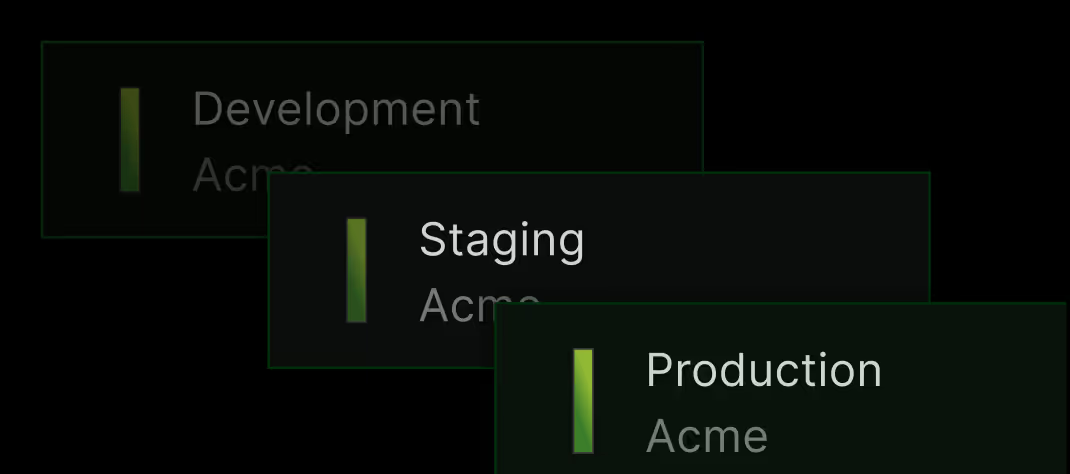
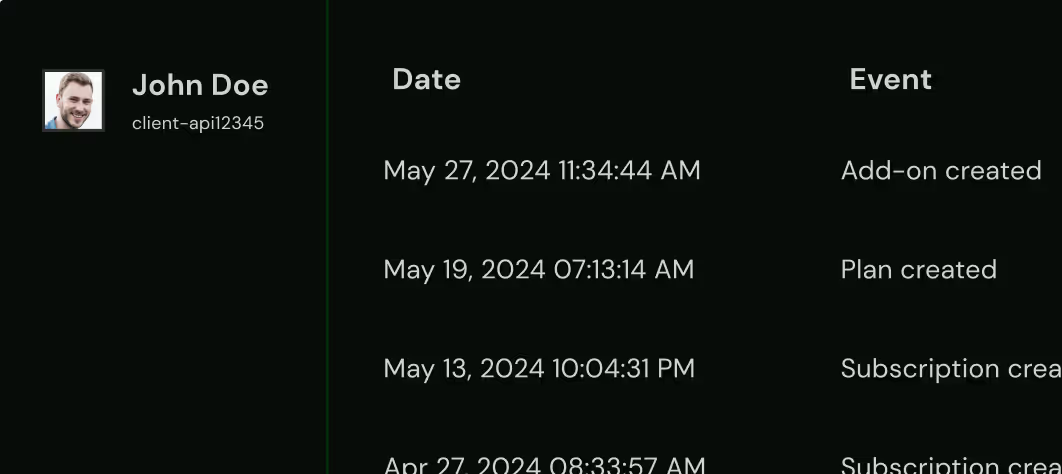


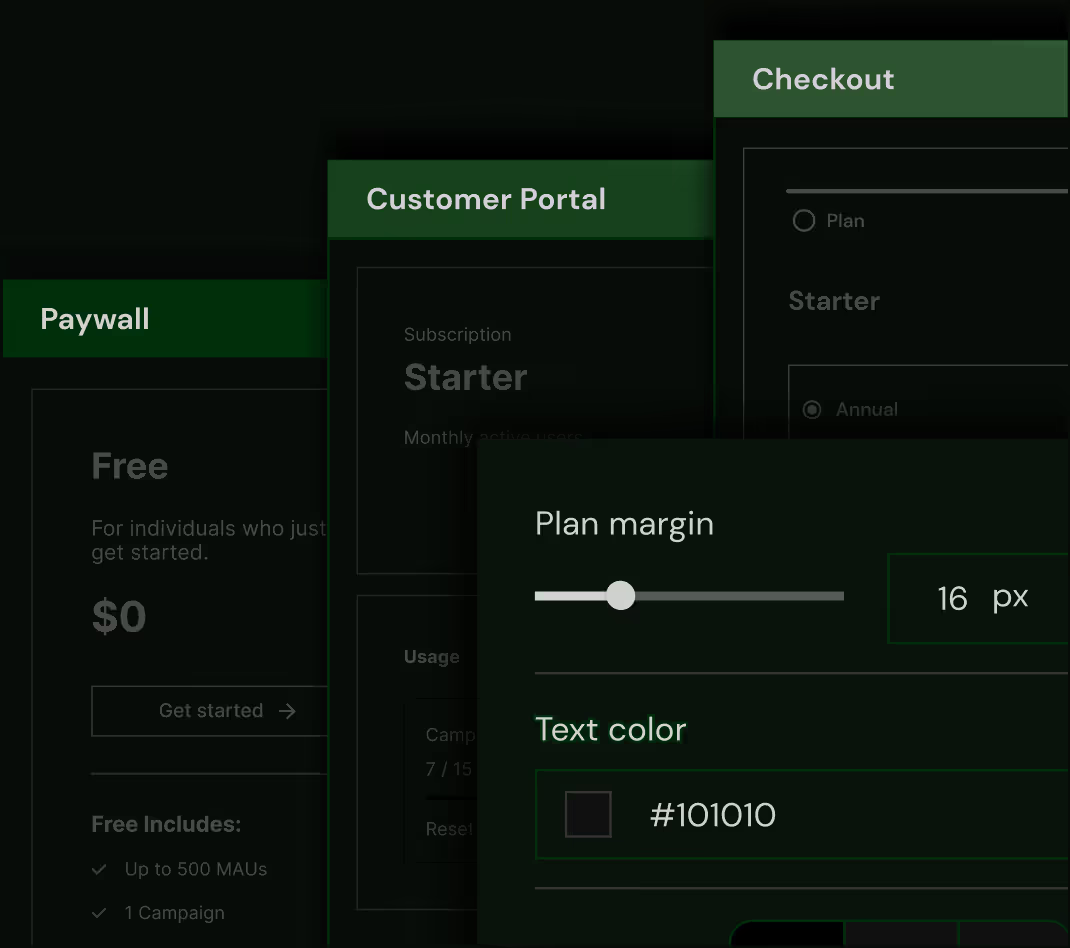
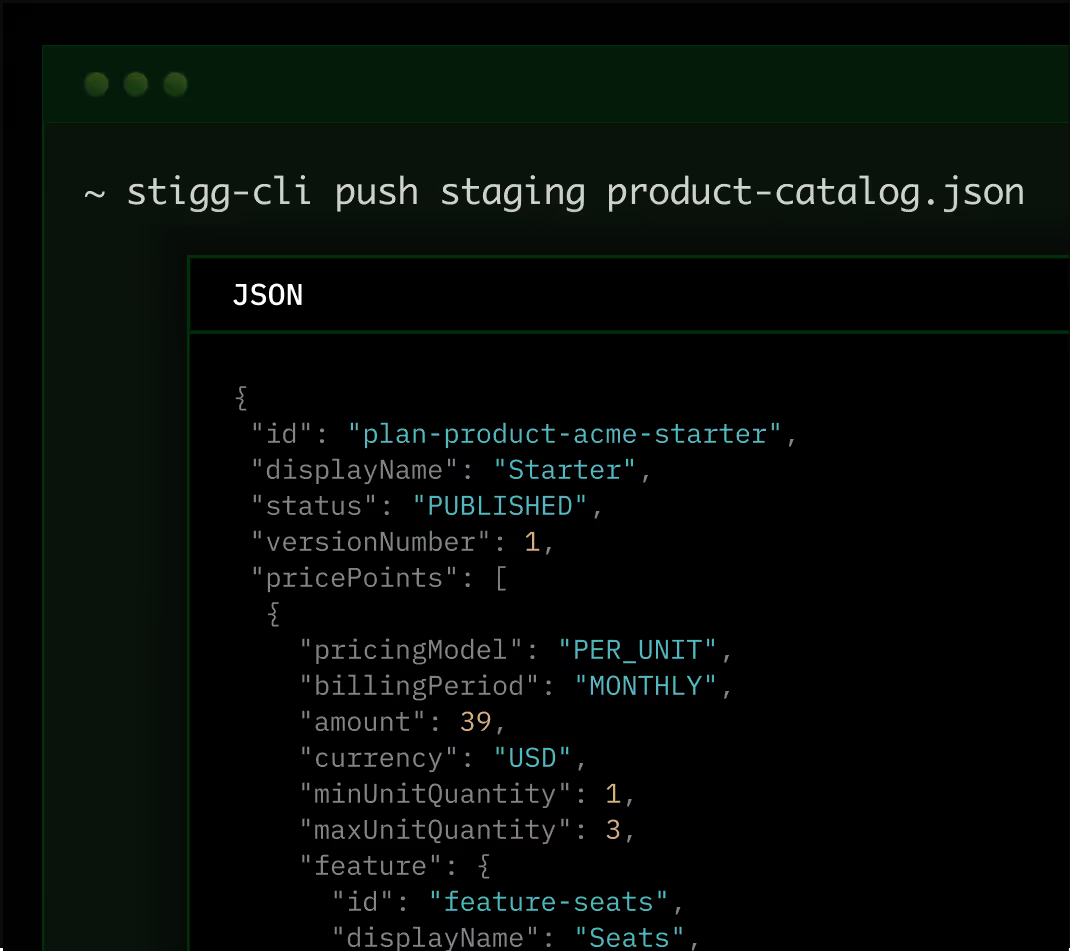
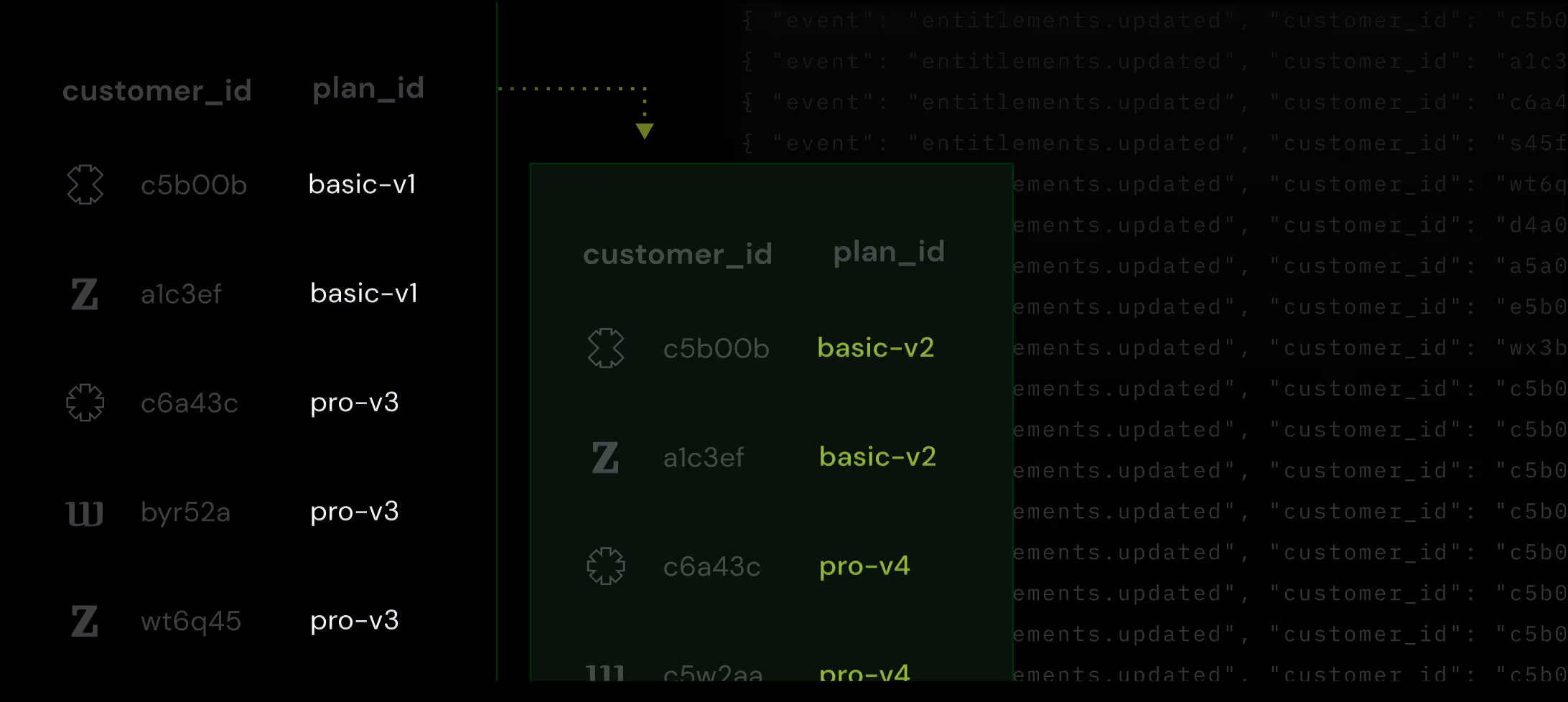


Built for speed & scale with an always-on architechture
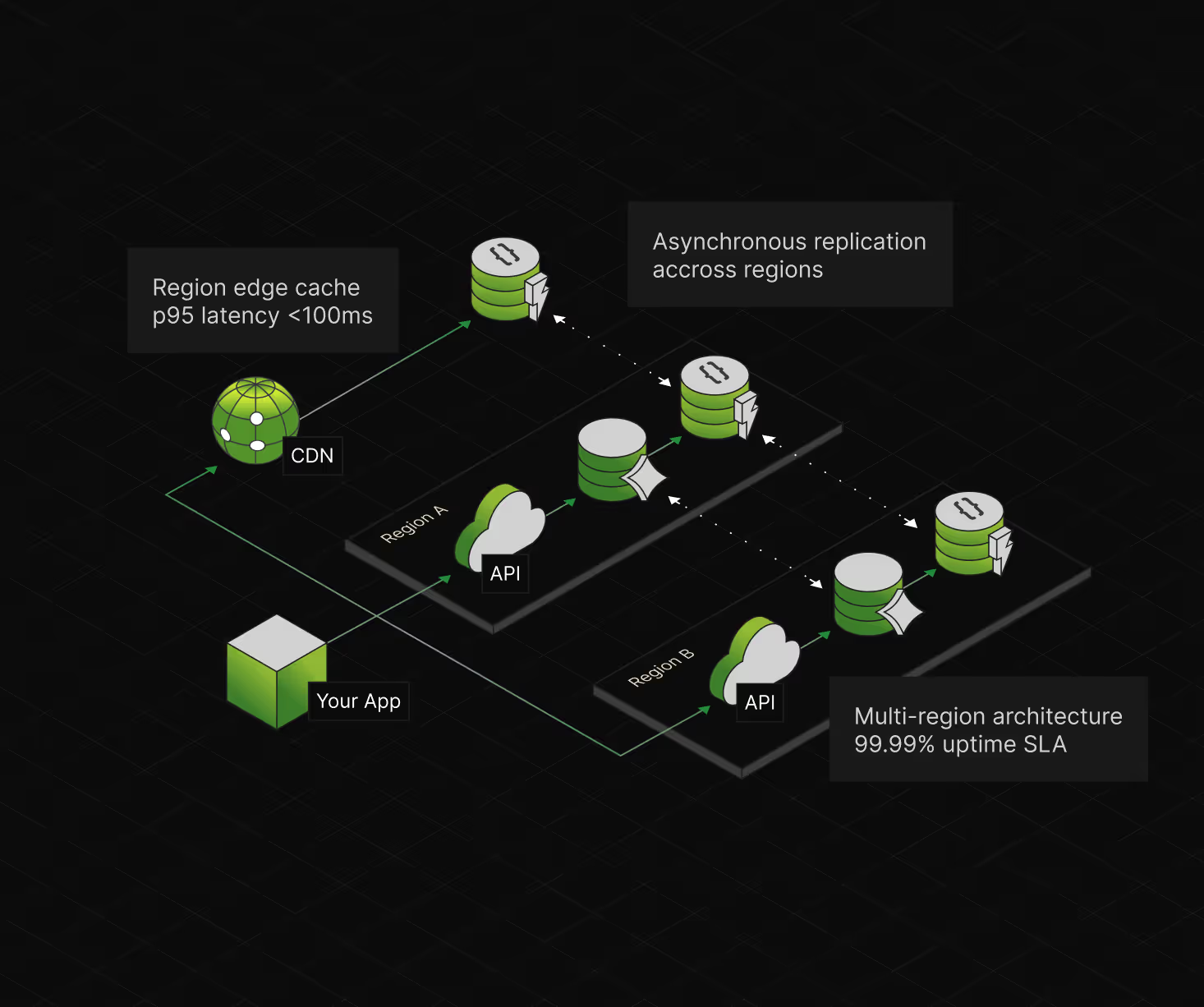


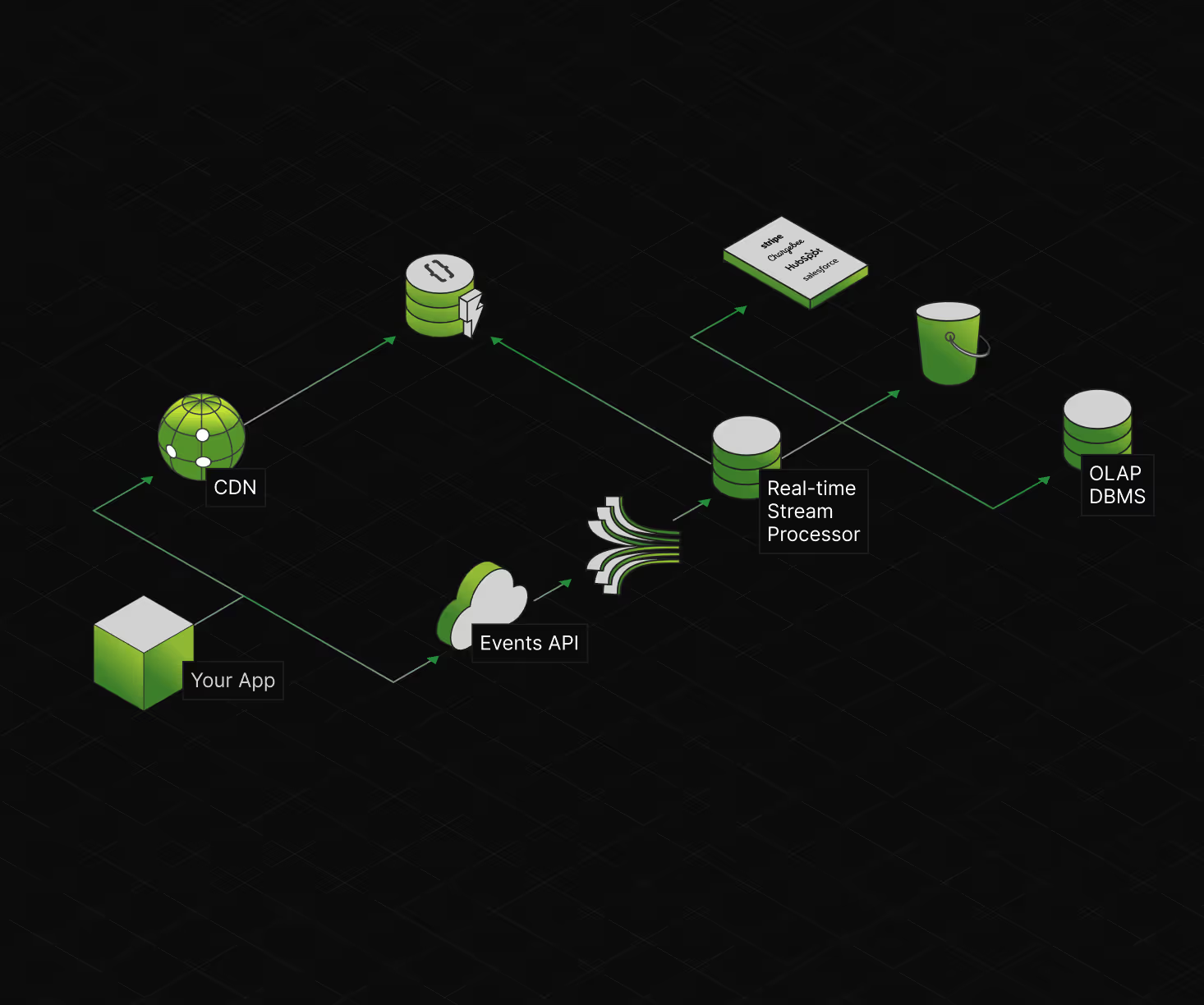

Over the last 1 month, all kinds of crazy ideas have been thrown at me and I’m like “yes we can do that”.
1yr ago, a request for addons came or just redoing our packaging and I gave a conservative timeline of 4 months.

Collaborating with the team at Stigg is great, they have deep knowledge of the SaaS monetization domain, and have been a great partner. With Stigg's product integrated, we plan to evolve our offering at a higher pace. Reducing the time to introduce new plans, price points or products from months to days.

We evaluated other options and selected Stigg as our Monetization platform, as it was the only solution that could support both the needs of AI21 Studio (B2B) and Wordtune (B2C) combined, while keep adding more options for us to price and package as we scale.

Flexibility in pricing & plans is critical for the success of SaaS companies. Even harder when catering for both Enterprise and PLG sales. You don't want these operational considerations to become an obstacle for success.

Not consulting, but if you're looking for a product to help with your PLG pricing, you should check out Stigg.
